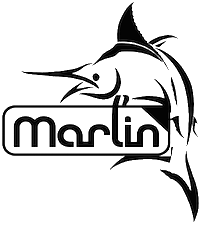
Period_seconds * 1000 may overflow in 16-bit architectures, resulting in a wraparound, thus a negative result. This negative integer, when interpreted as an unsigned long, it's a very large number. Thus the comparison may always yield false. Issue 1309: https://github.com/MarlinFirmware/Marlin/pull/1309"
Bug fixed by commit 30248214c7f
Type | IntegerOverflow |
Config | "THERMAL_RUNAWAY_PROTECTION_PERIOD && THERMAL_RUNAWAY_PROTECTION_PERIOD > 0" (2nd degree) |
Fix-in | code |
Location | temperature/ |
#include <stdio.h> unsigned long millis = 123456789l; #if defined (THERMAL_RUNAWAY_PROTECTION_PERIOD) && THERMAL_RUNAWAY_PROTECTION_PERIOD > 0 void thermal_runaway_protection(int *state, unsigned long *timer, float temperature, float target_temperature, int heater_id, int period_seconds, int hysteresis_degc) { if (temperature >= (target_temperature - hysteresis_degc)) { *timer = 123456782l; } else if ( (millis - *timer) > (period_seconds) * 1000) { printf("%s","Thermal Runaway, system stopped! Heater_ID: "); printf("%d",(int)heater_id); printf("%s","THERMAL RUNAWAY"); } } #endif int main(void){ thermal_runaway_protection(0, 123456783, 145.0f, 200.0f, 1, 10, 1); return 0; }
diff --git a/simple/3024821.c b/simple/3024821.c --- a/simple/3024821.c +++ b/simple/3024821.c @@ -8,7 +8,7 @@ if (temperature >= (target_temperature - hysteresis_degc)) { *timer = 123456782l; } - else if ( (millis - *timer) > (period_seconds) * 1000) + else if ( (millis - *timer) > (unsigned long)(period_seconds) * 1000) { printf("%s","Thermal Runaway, system stopped! Heater_ID: "); printf("%d",(int)heater_id);
#include <stdio.h> int main(void){ unsigned long millis = 123456789l; #if defined (THERMAL_RUNAWAY_PROTECTION_PERIOD) && THERMAL_RUNAWAY_PROTECTION_PERIOD > 0 int state = 0; unsigned long *timer = 123456782l; float temperature = 145.0f; float target_temperature = 200.0f; int heater_id = 1; int period_seconds = 10; int hysteresis_degc = 1; if (temperature >= (target_temperature - hysteresis_degc)) { *timer = 123456782; } else if ( (millis - *timer) > (period_seconds) * 1000) { printf("%s","Thermal Runaway, system stopped! Heater_ID: "); printf("%d",(int)heater_id); printf("%s","THERMAL RUNAWAY"); } #endif return 0; }
. call Marlin/temperature.cpp:1017: void thermal_runaway_protection(...) . ERROR temperature.cpp:1052: else if ( (millis() - *timer) > (period_seconds) * 1000) // Comparing an unsigned long with an integer...