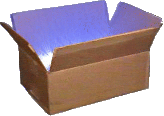
If CONFIG_FEATURE_HTTPD_GZIP is enabled and request contained 'Accept-Encoding: gzip', then errors were sent with 'Content-Encoding: gzip' even though they aren't."
Bug fixed by commit 95755181b82
Type | BehaviorViolation |
Config | BB_MMU && FEATURE_HTTPD_GZIP && FEATURE_HTTPD_BASIC_AUTH (3rd degree) |
Fix-in | code |
Location | networking/ |
#include <stdio.h> #include <stdlib.h> #include <string.h> #ifdef ENABLE_FEATURE_HTTPD_GZIP int content_gzip = 0; #endif #if ENABLE_FEATURE_HTTPD_BASIC_AUTH int authorized = 0; #endif #define HTTP_UNAUTHORIZED 401 #define IOBUF_SIZE 8192 char *iobuf = malloc(IOBUF_SIZE); void send_headers(int responseNum) { const char *mime_type; int len; /* error message is HTML */ mime_type = responseNum == HTTP_OK ? "application/octet-stream" : "text/html"; len = sprintf(iobuf, "HTTP/1.0 %d\r\nContent-type: %s\r\n", responseNum, mime_type); #ifdef ENABLE_FEATURE_HTTPD_BASIC_AUTH if (responseNum == HTTP_UNAUTHORIZED) { len += sprintf(iobuf + len, "WWW-Authenticate: Basic realm=\"Web Server Authentication\"\r\n"); } #endif if (content_gzip) len += sprintf(iobuf + len, "Content-Encoding: gzip\r\n"); printf("headers: '%s'\n", iobuf); } void send_headers_and_exit(int responseNum) { send_headers(responseNum); } void handle_incoming_and_exit() { #ifdef ENABLE_FEATURE_HTTPD_GZIP const char *s = strstr(iobuf, "gzip"); if (s) content_gzip = 1; #endif #ifdef ENABLE_FEATURE_HTTPD_BASIC_AUTH if(!authorized) send_headers_and_exit(HTTP_UNAUTHORIZED); #endif } #ifdef BB_MMU void mini_httpd() { handle_incoming_and_exit(); } #endif int main(int argc, char** argv) { #ifdef BB_MMU mini_httpd(); #endif return 0; }
diff --git a/simple/9575518.c b/simple/9575518.c --- a/simple/9575518.c +++ b/simple/9575518.c @@ -41,6 +41,9 @@ void send_headers_and_exit(int responseNum) { +#ifdef ENABLE_FEATURE_HTTPD_GZIP + content_gzip = 0; +#endif send_headers(responseNum); }
#include <stdio.h> #include <stdlib.h> #ifdef ENABLE_FEATURE_HTTPD_GZIP int content_gzip; #endif int main(int argc, char** argv) { // mini_httpd(); #ifdef ENABLE_FEATURE_HTTPD_GZIP if (rand() % 2) content_gzip = 1; #endif #ifdef ENABLE_FEATURE_HTTPD_BASIC_AUTH if (content_gzip) printf("Content-Encoding: gzip\r\n"); #endif return 0; }
. [BB_MMU] call networking/httpd.c:2458:mini_httpd() .. call 2242:handle_incoming_and_exit() ... [FEATURE_HTTPD_GZIP] 2095:content_gzip = 1; ... [FEATURE_HTTPD_BASIC_AUTH] call 2117:send_headers_and_exit() .... call 1068:send_headers() .... 1044:if(content_gzip){...}